We have just finished the initial release of our “Sign-in with Apple” cn1lib, which adds “Sign-in with Apple” support to Codename One apps. On iOS 13 and higher, this will use Apple’s native Authentication framework. On other platforms (e.g. Android, Desktop, and Simulator), this will use Apple’s Oauth2 authentication service.
The main motivation for adding this functionality is that Apple would require apps that use “sign in with…” to support its service too. If you won’t support sign in with Apple but include support signin with Facebook/Google your app might be rejected in the future.
If your app doesn’t require sign-in or uses custom login logic there’s no requirement to support “sign in with Apple” |
Getting Started
The hardest part of adding Apple sign-in support house-keeping you need to perform in Apple’s developer portal. If you only intend to support Apple sign-in with your iOS app, and not on other platforms, then the process is pretty simple – you just check a box next to “Sign-in with Apple” in the capabilites section of your App ID details page. Set-up for other platforms is a bit more involved. You need to create a “Services ID” (used for the Oauth2 client ID), and generate a private key so you will be able to generate the Oauth2 client secret on-demand.
For full instructions see the setup documentation in the cn1lib’s wiki.
You can find the plugin in Codename One Settings. Once you’ve added the cn1lib to your Codename One project, you can begin using the AppleLogin
class to provide Apple sign-in support.
The Code
The following is an example of how to add Apple login support to your app.
AppleLogin login = new AppleLogin();
// If using on non-iOS platforms, set Oauth2 settings here:
// login.setClientId(...);
// login.setKeyId(...);
// login.setTeamId(...);
// login.setRedirectURI(...);
// login.setPrivateKey(...);
if (login.isUserLoggedIn()) {
new MainForm().show();
} else {
new LoginForm().show();
}
....
class LoginForm extends Form {
LoginForm() {
super(BoxLayout.y());
$(getContentPane()).setPaddingMillimeters(3f, 0, 0, 0);
add(FlowLayout.encloseCenter(new Label(AppleLogin.createAppleLogo(0x0, 15f))));
Button loginBtn = new Button("Sign in with Apple");
AppleLogin.decorateLoginButton(loginBtn, 0x0, 0xffffff);
loginBtn.addActionListener(evt->{
login.doLogin(new LoginCallback() {
@Override
public void loginFailed(String errorMessage) {
System.out.println("Login failed");
ToastBar.showErrorMessage(errorMessage);
}
@Override
public void loginSuccessful() {
new MainForm().show();
}
});
});
add(FlowLayout.encloseCenter(loginBtn));
}
}
....
class MainForm extends Form {
MainForm() {
super(BoxLayout.y());
add(new SpanLabel("You are now logged in as "+login.getEmail()));
Button logout = new Button("Logout from Apple");
logout.addActionListener(e->{
login.doLogout();
new LoginForm().show();
});
add(logout);
}
}
For full working demo, see the Demo app
Some screenshots from the demo:
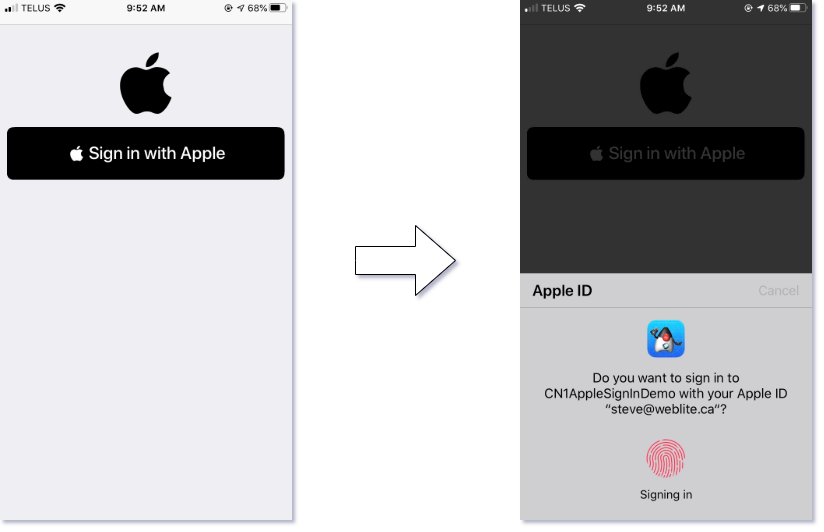
More information
-
See the Demo project for an example.
-
See the set-up instructions detailed instructions on adding support for Apple Sign-in.
3 Comments
This cn1 lib breaks the host app that imports it at compile time. The reason is that the native javase bit of the lib has incorrect package names and some imports aren’t available (in AppleSignInNativeImpl and WebViewBrowserWindow). The host app will compile when manually commenting these things out, but it might be worth updating the cn1lib so it doesn’t throw these errors as currently the native interface gets reset to faulty code each time the cn1lib is refreshed
Also, even after doing these things the following error is thrown on the server when compiling AppleLogin:
com_codename1_social_AppleLogin.m:22:10: fatal error: ‘java_io_StringReader.h’ file not found
“#”include “java_io_StringReader.h”
Could it be that java.io.StringReader was used instead of com.codename1.util .regex.StringReader?
I have replied inside the issue you opened in the issue tracker. https://github.com/codenameone/CodenameOne/issues/3068
The gist of it is that the build.xml file needs to be updated, and you need to run an update libs. This won’t be needed for long as the build.xml file will come updated with the next plugin update.
I’ll wait until the next update then as I don’t need this right now, was just toying with it in preparation of Apple making this a requirement (apparently that has been rolled back to June last I heard because of the coronavirus crisis)
Thanks for this